UART HAL¶
The UART HAL definitions is in dev_uart.h
, it provide interfaces for uart
driver to implement. Here is a diagram for the uart interface.
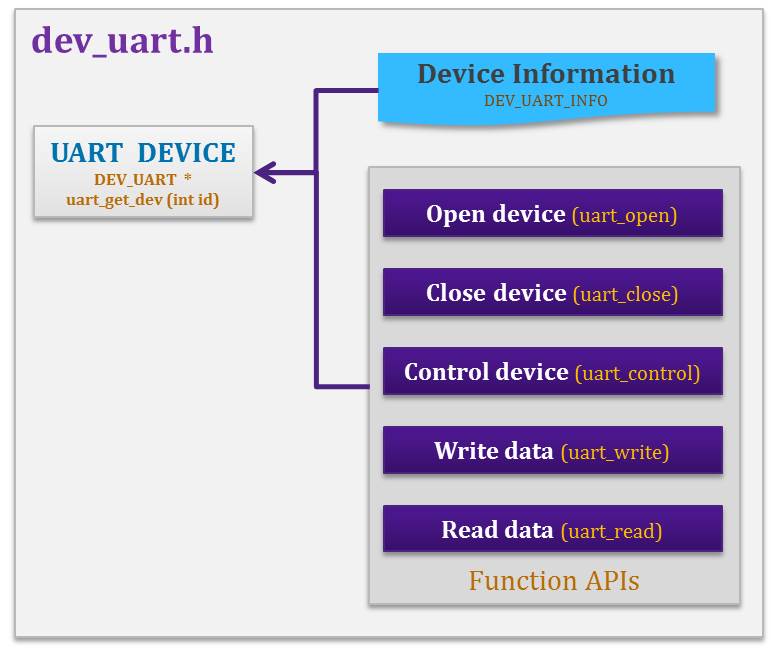
Definitions¶
-
group
DEVICE_HAL_UART_DEVSTRUCT
Contains definitions of uart device interface structure.
This structure will be used in user implemented code, which was called Device Driver Implement Layer for uart to use in implementation code. Application developer should use the UART API provided here to access to UART devices. BSP developer should follow the API definition to implement UART device drivers.
Defines
-
DEV_UART_INFO_SET_EXTRA_OBJECT
(uart_info_ptr, extra_info)¶ Set extra information pointer of uart info
-
DEV_UART_INFO_GET_EXTRA_OBJECT
(uart_info_ptr)¶ Get extra information pointer of uart info
Typedefs
-
typedef struct dev_uart_info
DEV_UART_INFO
¶ UART information struct definition.
informations about uart open count, working status, baudrate, uart registers and ctrl structure, uart dps format
-
typedef struct dev_uart_info *
DEV_UART_INFO_PTR
¶
Variables
-
int32_t (*
uart_open
)(uint32_t baud)¶ Open uart device
open an uart device with defined baudrate
- Parameters
baud – [in] initial baudrate of uart, must > 0
- Returns
E_OK – Open successfully without any issues
E_OPNED – If device was opened before with different parameters, then just increase the opn_cnt and return E_OPNED
E_OBJ – Device object is not valid
E_PAR – Parameter is not valid
E_NOSPT – Open settings are not supported
-
int32_t (*
uart_close
)(void)¶ Close uart device
close an uart device, just decrease the opn_cnt, if opn_cnt equals 0, then close the device
- Returns
E_OK – Close successfully without any issues(including scenario that device is already closed)
E_OPNED – Device is still opened, the device opn_cnt decreased by 1
E_OBJ – Device object is not valid
-
int32_t (*
uart_control
)(uint32_t ctrl_cmd, void *param)¶ Control uart device
control an uart device by ctrl_cmd, with passed param. you can control uart device using predefined uart control commands defined using DEV_SET_SYSCMD (which must be implemented by bsp developer), such as change baudrate, flush output and more. And you can also control uart device using your own specified commands defined using DEV_SET_USRCMD, but these specified commands should be defined in your own uart device driver implementation.
- Parameters
ctrl_cmd – [in] control command, to change or get some thing related to uart
param – [inout] parameters that maybe argument of the command, or return values of the command
- Returns
E_OK – Control device successfully
E_CLSED – Device is not opened
E_OBJ – Device object is not valid or not exists
E_PAR – Parameter is not valid for current control command
E_SYS – Control device failed, due to hardware issues, such as device is disabled
E_CTX – Control device failed, due to different reasons like in transfer state
E_NOSPT – Control command is not supported or not valid
-
int32_t (*
uart_write
)(const void *data, uint32_t len)¶ Send data by uart device(blocked)
send data through uart with defined len(blocked).
- Parameters
data – [in] pointer to data need to send by uart, must not be NULL
len – [in] length of data to be sent, must > 0
- Returns
>0 – Byte count that was successfully sent for poll method
E_OBJ – Device object is not valid or not exists
E_PAR – Parameter is not valid
E_SYS – Can’t write data to hardware due to hardware issues, such as device is disabled
-
int32_t (*
uart_read
)(void *data, uint32_t len)¶ Read data from uart device(blocked)
receive data of defined len through uart(blocked).
- Parameters
data – [out] pointer to data need to received by uart, must not be NULL
len – [in] length of data to be received, must > 0
- Returns
>0 – Byte count that was successfully received for poll method
E_OBJ – Device object is not valid or not exists
E_PAR – Parameter is not valid
E_SYS – Can’t receive data from hardware due to hardware issues, such as device is disabled
-
struct
dev_uart_info
¶ - #include <dev_uart.h>
UART information struct definition.
informations about uart open count, working status, baudrate, uart registers and ctrl structure, uart dps format
-
struct
dev_uart
¶ - #include <dev_uart.h>
UART device interface definition.
Define uart device interface, like uart information structure, provide functions to open/close/control uart, send/receive data by uart
Note
All this details are implemented by user in user porting code
-