SPI HAL¶
The SPI HAL definitions is in dev_spi.h
, it provide interfaces for spi
driver to implement. Here is a diagram for the spi interface.
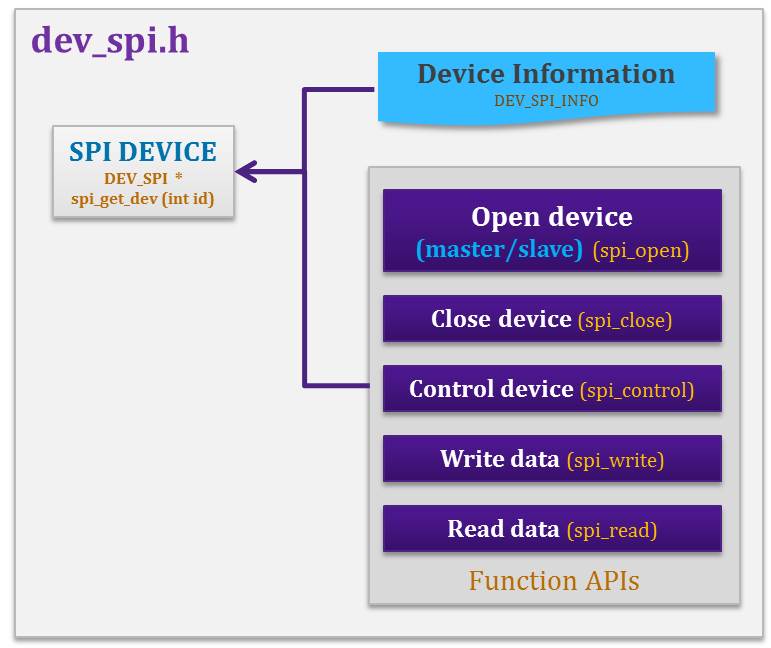
Definitions¶
-
group
DEVICE_HAL_SPI_DEVSTRUCT
contains definitions of spi device structure.
this structure will be used in user implemented code, which was called Device Driver Implement Layer for spi to realize in user code.
Defines
-
DEV_SPI_XFER_SET_TXBUF
(xfer, buf, ofs, len)¶ Set tx buffer of device spi transfer
-
DEV_SPI_XFER_SET_RXBUF
(xfer, buf, ofs, len)¶ Set rx buffer of device spi transfer
-
DEV_SPI_XFER_CALC_TOTLEN
(xfer)¶ Calculate total length of current transfer without next transfer
-
DEV_SPI_XFER_SET_NEXT
(xfer, next_xfer)¶ Set next SPI transfer
-
DEV_SPI_XFER_INIT
(xfer)¶ Init spi transfer
-
DEV_SPI_INFO_SET_EXTRA_OBJECT
(spi_info_ptr, extra_info)¶ Set extra information pointer of spi info
-
DEV_SPI_INFO_GET_EXTRA_OBJECT
(spi_info_ptr)¶ Get extra information pointer of spi info
-
SPI_DFS_DEFAULT
¶ Default spi data frame size
-
SPI_SLAVE_NOT_SELECTED
¶ Slave is not selected
-
SPI_DUMMY_DEFAULT
¶ default dummy value for first open
Typedefs
-
typedef struct dev_spi_transfer
DEV_SPI_TRANSFER
¶
-
typedef struct dev_spi_transfer *
DEV_SPI_TRANSFER_PTR
¶
-
typedef struct DEV_SPI_PAK *
DEV_SPI_PAK_PTR
¶
-
typedef struct dev_spi_info
DEV_SPI_INFO
¶ spi information struct definition
informations about spi open state, working state, frequency, spi registers, working method, interrupt number
-
typedef struct dev_spi_info *
DEV_SPI_INFO_PTR
¶
Variables
-
int32_t (*
spi_open
)(uint32_t mode, uint32_t param)¶ open spi device in master/slave mode, \ when in master mode, param stands for frequency, \ when in slave mode, param stands for clock mode
open an spi device with selected mode (master or slave) with defined param
- Parameters
mode – [in] working mode (master or slave)
param – [in] When mode is DEV_MASTER_MODE, param stands for frequency, when mode is DEV_SLAVE_MODE, param stands for slave clock mode
- Returns
E_OK – Open successfully without any issues
E_OPNED – If device was opened before with different parameters, then just increase the opn_cnt and return E_OPNED
E_OBJ – Device object is not valid
E_SYS – Device is opened for different mode before, if you want to open it with different mode, you need to fully close it first.
E_PAR – Parameter is not valid
E_NOSPT – Open settings are not supported
-
int32_t (*
spi_close
)(void)¶ close spi device
close an spi device, just decrease the opn_cnt, if opn_cnt equals 0, then close the device
- Returns
E_OK – Close successfully without any issues(including scenario that device is already closed)
E_OPNED – Device is still opened, the device opn_cnt decreased by 1
E_OBJ – Device object is not valid
-
int32_t (*
spi_control
)(uint32_t ctrl_cmd, void *param)¶ control spi device
control an spi device by ctrl_cmd, with passed param. you can control spi device using predefined spi control commands defined using DEV_SET_SYSCMD (which must be implemented by bsp developer), such as set spi master frequency, flush tx and more. And you can also control spi device using your own specified commands defined using DEV_SET_USRCMD, but these specified commands should be defined in your own spi device driver implementation.
- Parameters
ctrl_cmd – [in] control command, to change or get some thing related to spi
param – [inout] parameters that maybe argument of the command, or return values of the command, must not be NULL
- Returns
E_OK – Control device successfully
E_CLSED – Device is not opened
E_OBJ – Device object is not valid or not exists
E_PAR – Parameter is not valid for current control command
E_SYS – Control device failed, due to hardware issues, such as device is disabled
E_CTX – Control device failed, due to different reasons like in transfer state
E_NOSPT – Control command is not supported or not valid
-
int32_t (*
spi_write
)(const void *data, uint32_t len)¶ send data to spi device (blocking method)
send data through spi with defined len to slave device .
- Parameters
data – [in] pointer to data need to send by spi
len – [in] length of data to be sent
- Returns
>0 – Byte count that was successfully sent for poll method
E_OBJ – Device object is not valid or not exists
E_PAR – Parameter is not valid
E_CTX – Device is still in transfer state
E_SYS – Can’t write data to hardware due to hardware issues, such as device is disabled
-
int32_t (*
spi_read
)(void *data, uint32_t len)¶ read data from spi device (blocking method)
receive data of defined len through spi from slave device .
- Parameters
data – [out] pointer to data need to received by spi
len – [in] length of data to be received
- Returns
>0 – Byte count that was successfully received for poll method
E_OBJ – Device object is not valid or not exists
E_CTX – Device is still in transfer state
E_PAR – Parameter is not valid
E_SYS – Can’t receive data from hardware due to hardware issues, such as device is disabled
-
struct
dev_spi_transfer
¶ - #include <dev_spi.h>
spi read and write data structure used by SPI_CMD_TRANSFER_INT or SPI_CMD_TRANSFER_POLLING spi write then read data
-
struct
DEV_SPI_PAK
¶ - #include <dev_spi.h>
spi read and write data structure used by SPI_CMD_QUAD_READ spi write cmd and address then read data
-
struct
dev_spi_info
¶ - #include <dev_spi.h>
spi information struct definition
informations about spi open state, working state, frequency, spi registers, working method, interrupt number
-
struct
dev_spi
¶ - #include <dev_spi.h>
spi device interface definition
define spi device interface, like spi information structure, fuctions to get spi info, open/close/control spi, send/receive data by spi
Note
all this details are implemented by user in user porting code
-