I2C HAL¶
The I2C HAL definitions is in dev_i2c.h
, it provide interfaces for i2c
driver to implement. Here is a diagram for the i2c interface.
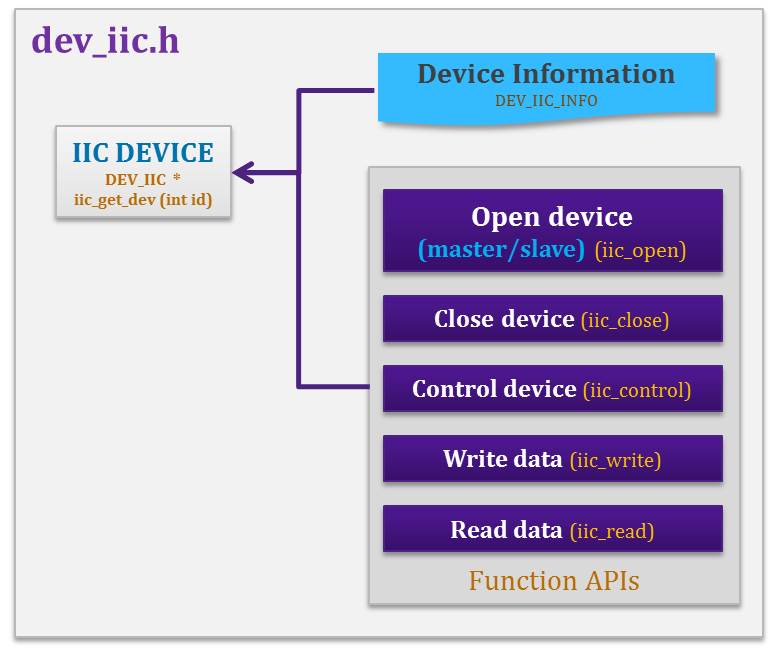
Definitions¶
-
group
DEVICE_HAL_IIC_DEVSTRUCT
contains definitions of iic device structure.
this structure will be used in user implemented code, which was called Device Driver Implement Layer for iic to realize in user code.
Defines
-
DEV_IIC_INFO_SET_EXTRA_OBJECT
(iic_info_ptr, extra_info)¶ Set extra information pointer of iic info
-
DEV_IIC_INFO_GET_EXTRA_OBJECT
(iic_info_ptr)¶ Get extra information pointer of iic info
Typedefs
-
typedef struct dev_iic_info
DEV_IIC_INFO
¶ iic information struct definition
informations about iic open state, working state, baurate, iic registers, working method, interrupt number
-
typedef struct dev_iic_info *
DEV_IIC_INFO_PTR
¶
Variables
-
int32_t (*
iic_open
)(uint32_t mode, uint32_t param)¶ open iic device in master/slave mode, \ when in master mode, param stands for speed mode, \ when in slave mode, param stands for slave address
open an iic device with selected mode (master or slave) with defined param
- Parameters
mode – [in] working mode (master or slave)
param – [in] When mode is DEV_MASTER_MODE, param stands for speed mode, when mode is DEV_SLAVE_MODE, param stands for slave device 7bit address
- Returns
E_OK – Open successfully without any issues
E_OPNED – If device was opened before with different parameters, then just increase the opn_cnt and return E_OPNED
E_OBJ – Device object is not valid
E_SYS – Device is opened for different mode before, if you want to open it with different mode, you need to fully close it first.
E_PAR – Parameter is not valid
E_NOSPT – Open settings are not supported
-
int32_t (*
iic_close
)(void)¶ close iic device
close an iic device, just decrease the opn_cnt, if opn_cnt equals 0, then close the device
- Returns
E_OK – Close successfully without any issues(including scenario that device is already closed)
E_OPNED – Device is still opened, the device opn_cnt decreased by 1
E_OBJ – Device object is not valid
-
int32_t (*
iic_control
)(uint32_t ctrl_cmd, void *param)¶ control iic device
control an iic device by ctrl_cmd, with passed param. you can control iic device using predefined iic control commands defined using DEV_SET_SYSCMD (which must be implemented by bsp developer), such as set iic speed mode, flush tx and more. And you can also control iic device using your own specified commands defined using DEV_SET_USRCMD, but these specified commands should be defined in your own iic device driver implementation.
- Parameters
ctrl_cmd – [in] control command, to change or get some thing related to iic
param – [inout] parameters that maybe argument of the command, or return values of the command, must not be NULL
- Returns
E_OK – Control device successfully
E_CLSED – Device is not opened
E_OBJ – Device object is not valid or not exists
E_PAR – Parameter is not valid for current control command
E_SYS – Control device failed, due to hardware issues, such as device is disabled
E_CTX – Control device failed, due to different reasons like in transfer state
E_NOSPT – Control command is not supported or not valid
-
int32_t (*
iic_write
)(const void *data, uint32_t len)¶ send data by iic device (blocking method)
send data through iic with defined len to slave device which slave address is slv_addr.
- Parameters
data – [in] pointer to data need to send by iic
len – [in] length of data to be sent
- Returns
>0 – Byte count that was successfully sent for poll method, it might can’t send that much due to different error state.
E_OBJ – Device object is not valid or not exists
E_PAR – Parameter is not valid
E_CTX – Device is still in transfer state
E_SYS – Can’t write data to hardware due to hardware issues, such as device is disabled
-
int32_t (*
iic_read
)(void *data, uint32_t len)¶ read data from iic device (blocking method)
receive data of defined len through iic from slave device which slave address is slv_addr.
- Parameters
data – [out] pointer to data need to received by iic
len – [in] length of data to be received
- Returns
>0 – Byte count that was successfully received for poll method, it might can’t send that much due to different error state.
E_OBJ – Device object is not valid or not exists
E_CTX – Device is still in transfer state
E_PAR – Parameter is not valid
E_SYS – Can’t receive data from hardware due to hardware issues, such as device is disabled
-
struct
dev_iic_info
¶ - #include <dev_iic.h>
iic information struct definition
informations about iic open state, working state, baurate, iic registers, working method, interrupt number
-
struct
dev_iic
¶ - #include <dev_iic.h>
iic device interface definition
define iic device interface, like iic information structure, fuctions to get iic info, open/close/control iic, send/receive data by iic
Note
all this details are implemented by user in user porting code
-